While loop is also known as a pre-tested loop. It repeatedly executes a target statement as long as a given condition is true. It can be viewed as a repeating if statement.
The while loop is mostly used in the case where the number of iterations is not known in advance.
Syntax
while(condition) {
statement(s);
}
- The condition may be any expression, and true is any nonzero value.
- The loop iterates while the condition is true.
- When the condition becomes false, the program control passes to the line immediately following the loop.
How while loop works?
- The
while
loop evaluates theCondition
inside the parentheses()
. - If
Condition
is true, statements inside the body ofwhile
loop are executed. Then,Condition
is evaluated again. - The process goes on until
Condition
is evaluated to false. - If
Condition
is false, the loop terminates (ends).
Flowchart of while loop in C
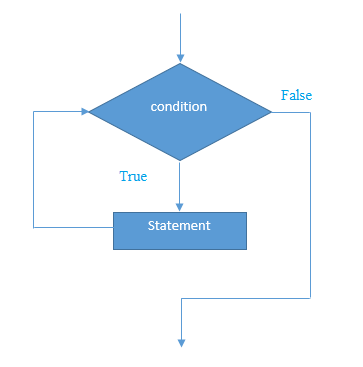
// Print numbers from 1 to 5
#include <stdio.h>
int main() {
int i = 1;
while (i <= 5) {
printf("%d\n", i);
++i;
}
return 0;
}
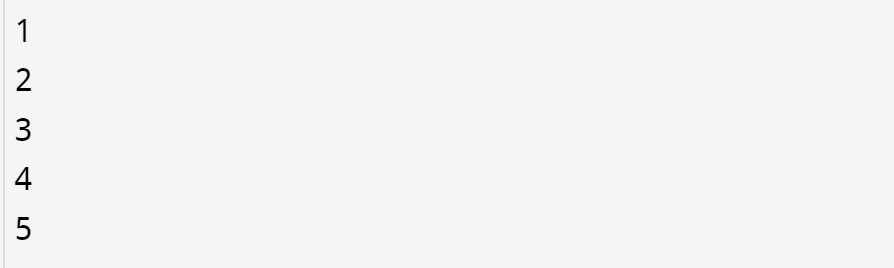