The switch statement in C is an alternate to if-else-if ladder statement. It allows a variable to be tested for equality against a list of values. Each value is called a case.
Basically, it is used to perform different actions based on different conditions(cases).
In this tutorial, you will learn to create the switch statement in C programming with the help of an example.
Syntax of switch…case
switch(expression) {
case constant-expression :
statement(s);
break; /* optional */
case constant-expression :
statement(s);
break; /* optional */
/* you can have any number of case statements */
default : /* Optional */
statement(s);
}
The expression is evaluated once and compared with the values of each case label. If there is a match, the corresponding statements after the matching label are executed until break
is encountered.
You can have any number of case statements within a switch. Each case is followed by the value to be compared to and a colon.
When the variable being switched on is equal to a case, the statements following that case will execute until a break statement is reached.
The break statement in switch case is not must. It is optional.
If there is no break statement found in the case, all the cases will be executed present after the matched case. It is known as fall through the state of C switch statement.
A switch statement can have an optional default case, which must appear at the end of the switch.
The default case can be used for performing a task when none of the cases is true.
No break is needed in the default case.
Rules for switch statement in C language
The constant-expression for a case must be the same data type as the variable in the switch, and it must be a constant or a literal.
The switch expression must be of an integer or character type.
The case value can be used only inside the switch statement.
Some examples of valid and invalid switch case. Consider the following variables.
int x,y,z;
char a,b;
float f;
Valid Switch | Invalid Switch | Valid Case | Invalid Case |
---|---|---|---|
switch(x) | switch(f) | case 3; | case 2.5; |
switch(x>y) | switch(x+2.5) | case ‘a’; | case x; |
switch(a+b-2) | case 1+2; | case x+2; | |
switch(func(x,y)) | case ‘x’>’y’; | case 1,2,3; |
Flowchart of switch statement in C
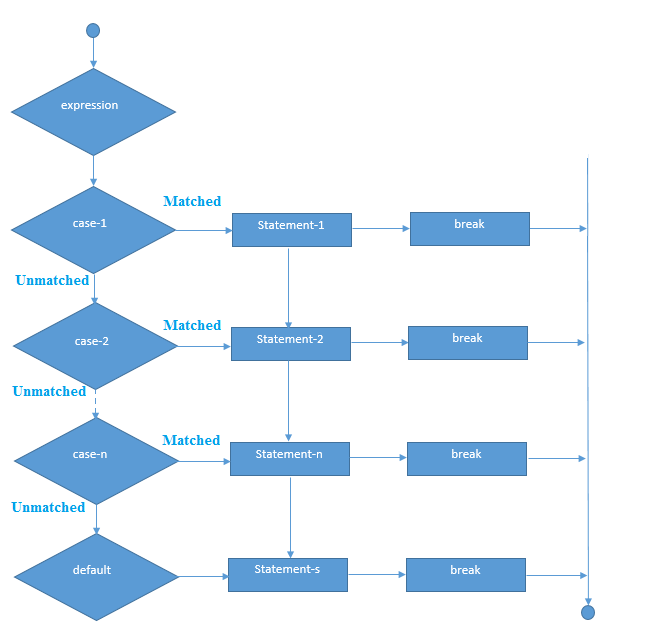
Example
#include <stdio.h>
int main () {
/* local variable definition */
char grade = 'B';
switch(grade) {
case 'A' :
printf("Excellent!\n" );
break;
case 'B' :
case 'C' :
printf("Well done\n" );
break;
case 'D' :
printf("You passed\n" );
break;
case 'F' :
printf("Better try again\n" );
break;
default :
printf("Invalid grade\n" );
}
printf("Your grade is %c\n", grade );
return 0;
}
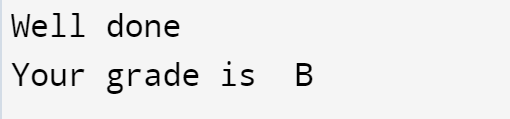