The break is a keyword in C. It is used to bring the program control out of the loop. In C, if you want to exit a loop when a specific condition is met, you can use the break
statement.
The break statement is used inside loops or switch statement. It can be used to terminate a case in the switch statement.
The break statement ends the loop immediately when it is encountered. When a break statement is encountered inside a loop, the loop is immediately terminated and the program control resumes at the next statement following the loop.
In this tutorial, you will learn to use break statement with the help of examples.
Syntax
break;
Flowchart
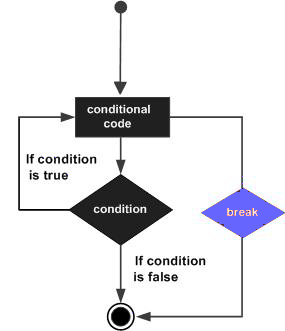
The break statement is almost always used with if...else
statement inside the loop.
Example
#include <stdio.h>
int main () {
/* local variable definition */
int a = 10;
/* while loop execution */
while( a < 20 ) {
printf("value of a: %d\n", a);
a++;
if( a > 15) {
/* terminate the loop using break statement */
break;
}
}
return 0;
}
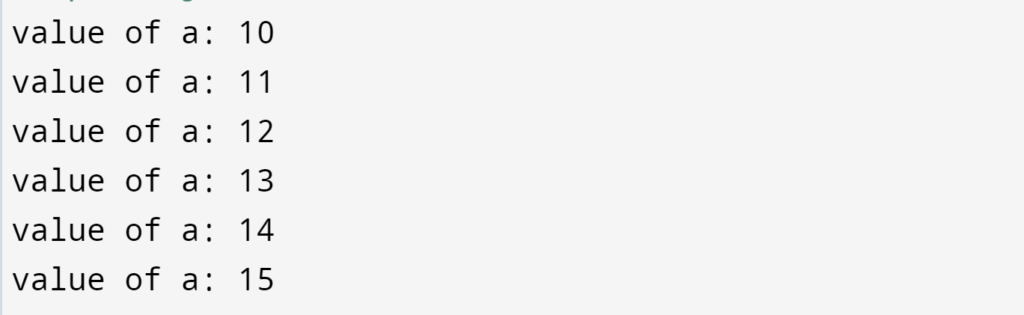
C break statement with the nested loop
If you are using nested loops, the break statement will stop the execution of the innermost loop and start executing the next line of code after the block.
Example
// C program to illustrate
// using break statement
// in Nested loops
#include <stdio.h>
int main() {
// nested for loops with break statement
// at inner loop
for (int i = 0; i < 5; i++) {
for (int j = 1; j <= 10; j++) {
if (j > 3)
break;
else
printf("*");
}
printf("\n");
}
return 0;
}
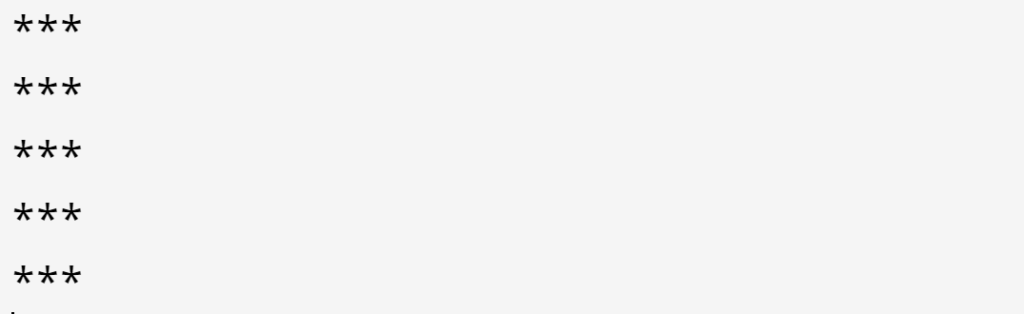