The if-else statement is used to perform two operations for a single condition. Here if statement has an optional else block, which will execute when the Boolean expression is false.
The if-else statement is an extension to the if statement using which, we can perform two different operations, i.e., one is for the correctness of that condition, and the other is for the incorrectness of the condition.
Syntax
if(boolean_expression) {
/* statement(s) will execute if the boolean expression is true */
} else {
/* statement(s) will execute if the boolean expression is false */
}
- If the boolean_expression is evaluated to true, statements inside the body of
if
are executed. - If the boolean_expression is evaluated to false, statements inside the body of
else
are executed.
Flowchart
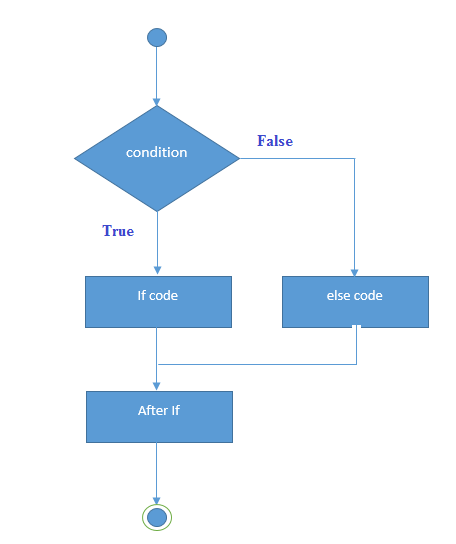
Example
#include<stdio.h>
int main(){
int number=0;
printf("enter a number:");
scanf("%d",&number);
if(number%2==0){
printf("%d is even number",number);
}
else{
printf("%d is odd number",number);
}
return 0;
}
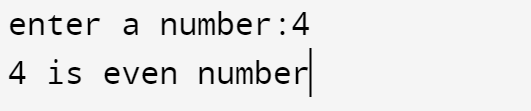
C programming language assumes any non-zero and non-null values as true, and if it is either zero or null, then it is assumed as false value.