One Dimensional or 1-D Array in C with Examples. One dimensional array is an array that has only one subscript specification that is needed to specify a particular element of an array.
An array variable must be declared before being used in a program.
An array index always starts from 0. For example, if an array variable is declared as s[10], then it ranges from 0 to 9.
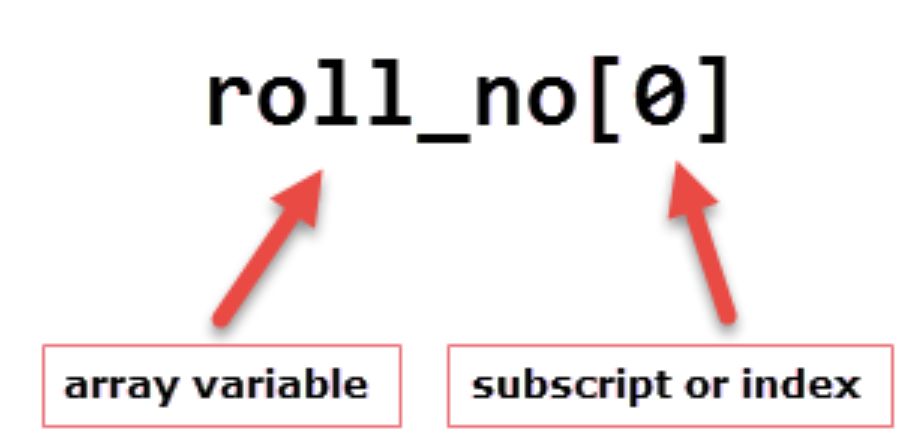
Arrays can be single or multidimensional.
The number of subscript or index determines the dimensions of the array.
You can also say that one-dimensional or 1-D array is a structured collection of components also called array elements that can be accessed individually by specifying the position of a component with a single index value.
Declaration of 1-D Array in C Language
data_type array_name[array_size];
Example
int marks[5]; //Here, int is the data_type, marks are the array_name, and 5 is the array_size.
Initialization of One-Dimensional Array in C
You can initialize an array by using the index of each element.
An array can be initialized in two ways, which are as follows −
- Compile time initialization – Also known as static initialization.
- Runtime initialization
Example – Compile time initialization
The compile-time initialization means the array of the elements are initialized at the time the program is written or array declaration.
marks[0]=80;//initialization of array
marks[1]=60;
marks[2]=70;
marks[3]=85;
marks[4]=75;
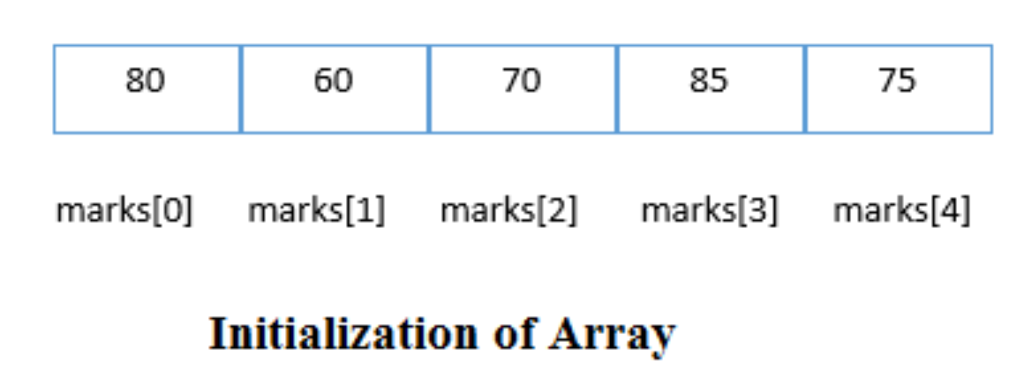
Declaration with Initialization
int marks[5]={20,30,40,50,60}; //In such case, there is no requirement to define the size.
int marks1[]={20,30,40,50,60}; //Valid
Example – Run time initialization
Run time initialization means the array can be initialized at runtime. Here array elements are initialized after the compilation of the program.
#include<stdio.h>
main ( ){
int a[5],i;
printf ("enter 5 elements");
for ( i=0; i<5; i++)
scanf("%d", &a[i]);
printf("elements of the array are");
for (i=0; i<5; i++)
printf("%d", a[i]);
}
Example
#include<stdio.h>
int main(){
int i=0;
int marks[5]={20,30,40,50,60};//declaration and initialization of array
//traversal of array
for(i=0;i<5;i++){
printf("%d \n",marks[i]);
}
return 0;
}
Conceptually you can think of a one-dimensional array as a row, where elements are stored one after another.
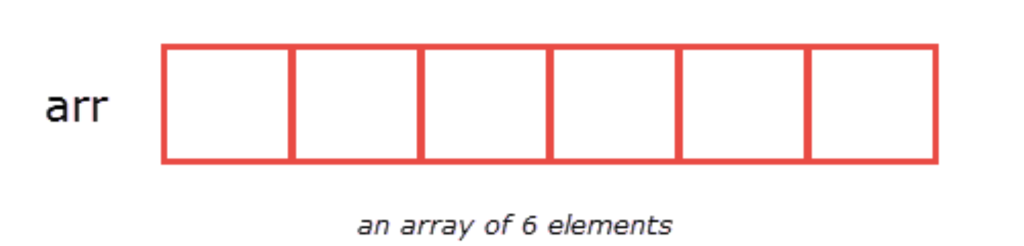