The goto statement is known as jump statement in C. It is used to transfer the program control to a predefined label.
The goto statement can be used to repeat some part of the code for a particular condition.
In this tutorial, you will learn to create the goto statement in C programming.
It can also be used to break the multiple loops which can’t be done by using a single break statement.
Syntax
label:
//some part of the code;
goto label;
The label is an identifier. It can be any plain text except C keyword and it can be set anywhere in the C program above or below to goto statement.
When the goto
statement is encountered, the control of the program jumps to label:
and starts executing the code.
Flowchart
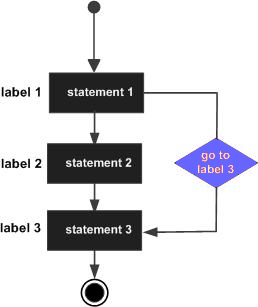
Example
#include <stdio.h>
int main() {
int num, i = 1;
printf("Enter the number whose table you want to print?");
scanf("%d", & num);
table:
printf("%d x %d = %d\n", num, i, num * i);
i++;
if (i <= 10)
goto table;
}
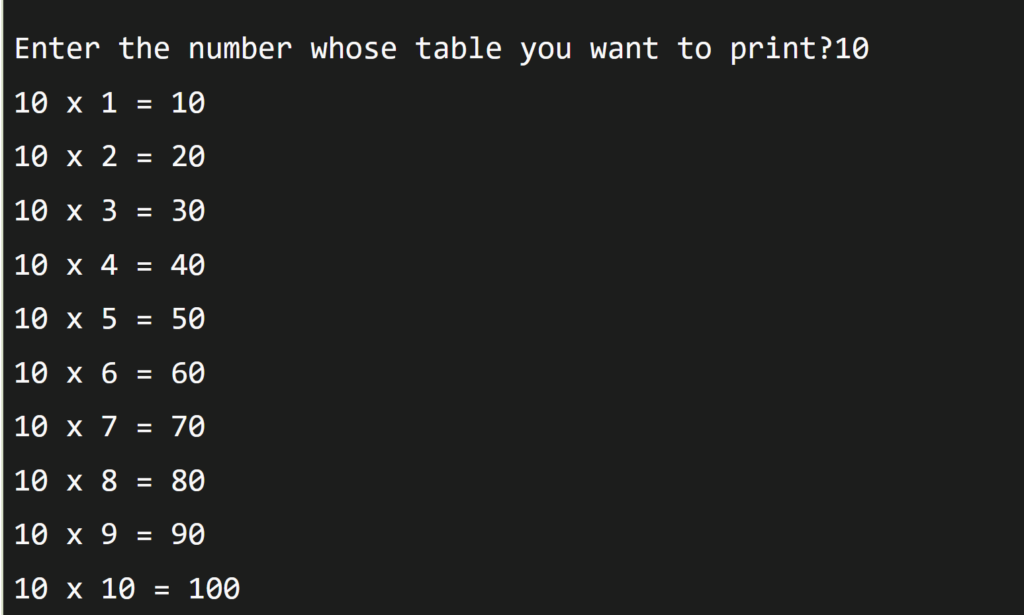
In the following example goto statement is used to break the multiple loops using a single statement at the same time.
#include <stdio.h>
int main() {
int i, j, k;
for (i = 0; i < 10; i++) {
for (j = 0; j < 5; j++) {
for (k = 0; k < 3; k++) {
printf("%d %d %d\n", i, j, k);
if (j == 3) {
goto out;
}
}
}
}
out:
printf("came out of the loop");
}
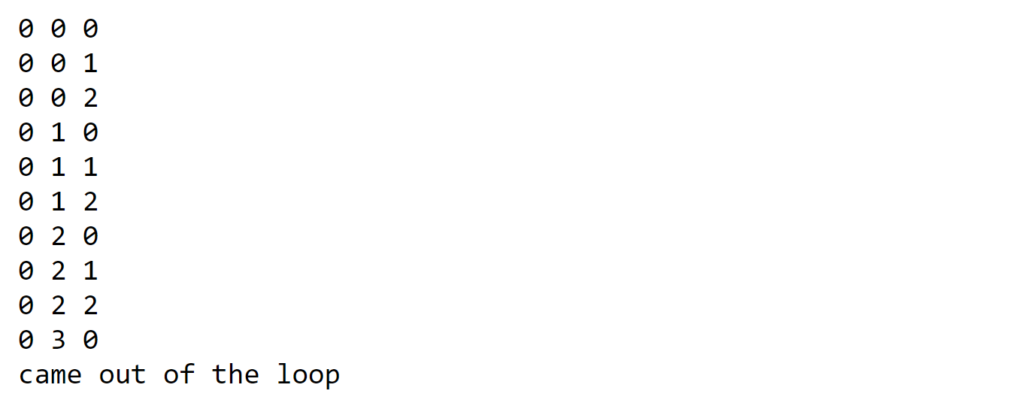