The do..while
loop is similar to the while
loop with one important difference that it is guaranteed to execute at least one time.
The do-while loop is mainly used in the case where we need to execute the loop at least once.
The body of do...while
loop is executed at least once. The do while loop is a post tested loop.
Contents
hide
Syntax
do {
statement(s);
} while( condition/expression );
The expression in a do-while statement is evaluated after the body of the loop is executed. Therefore, the body of the loop is always executed at least once.
How do while loop works?
- The statement body is executed.
- Next, expression is evaluated.
- If expression is false, the do-while statement terminates and control passes to the next statement in the program.
- If expression is true (nonzero), the process is repeated, beginning with step 1.
- This process repeats until the given condition becomes false.
Flowchart
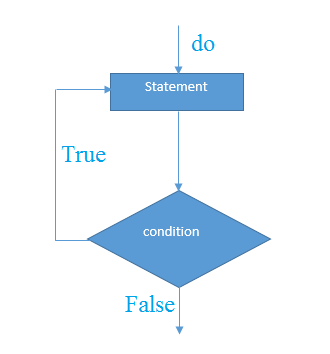
Example
#include <stdio.h>
int main () {
/* local variable definition */
int a = 10;
/* do loop execution */
do {
printf("value of a: %d\n", a);
a = a + 1;
}while( a < 20 );
return 0;
}
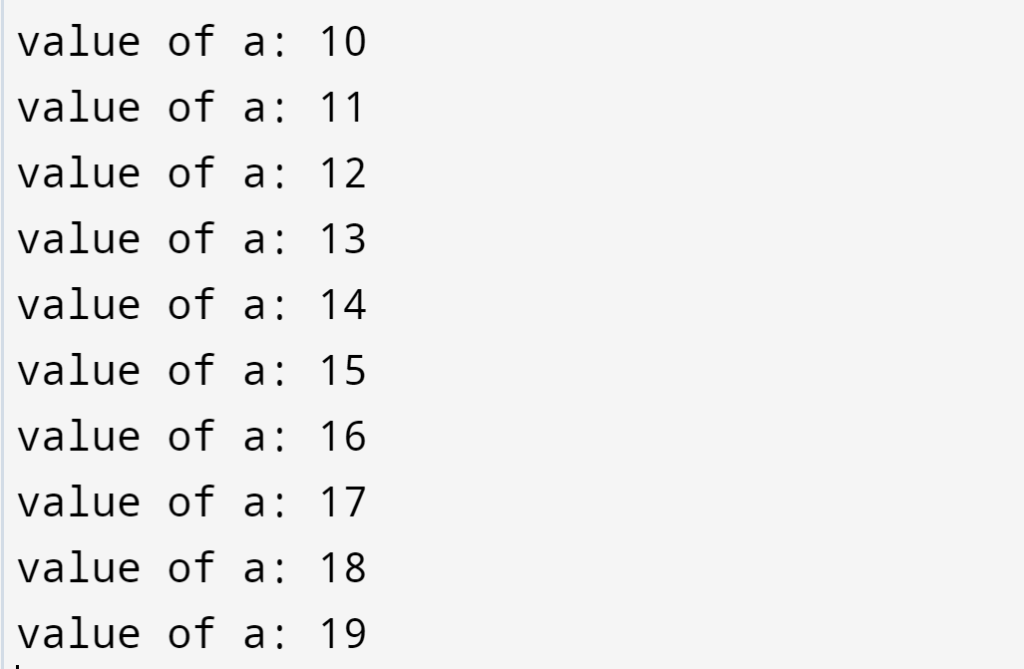