PHP if else statement is used to test condition. PHP If Else statements are also known as conditional statements.
Types of if else in PHP
- if
- if-else
- if-else-if
- nested if
PHP If Statement
It executes code if specified condition is true.
Syntax
if (condition) {
code to be executed if condition is true;
}
Flowchart
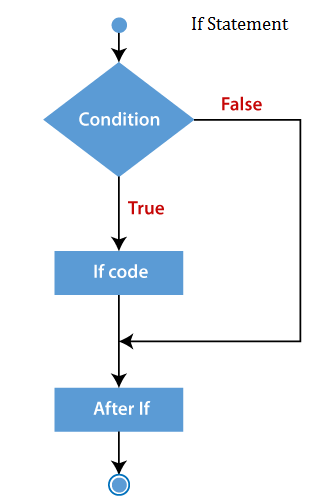
Example
<?php
$num=10;
if($num<100){
echo "$num is less than 100";
}
?>
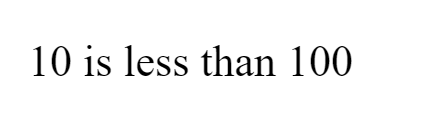
PHP If-else Statement
If-else statement is slightly different from if statement. It executes “if block” code if specified condition is true and “else block” code if specified condition is false.
PHP if-else statement is executed whether condition is true or false. It executes one block of code if the specified condition is true and another block of code if the condition is false.
Syntax
if (condition) {
code to be executed if condition is true;
} else {
code to be executed if condition is false;
}
Flowchart
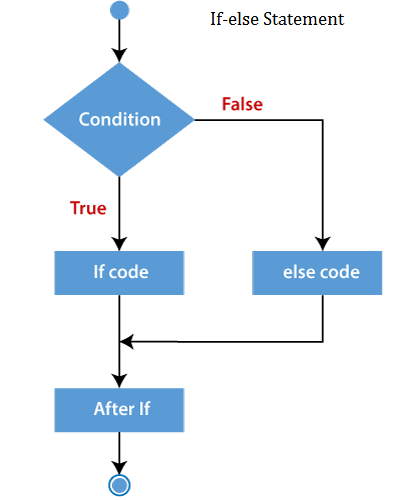
Example
<?php
$num=6;
if($num%2==0){
echo "$num is even number";
}else{
echo "$num is odd number";
}
?>
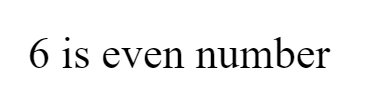
PHP If-else-if Statement
It is used to check multiple conditions. The if...elseif...else
statement executes different codes for more than two conditions.
Syntax
if (condition1){
//code to be executed if condition1 is true
} elseif (condition2){
//code to be executed if condition2 is true
} elseif (condition3){
//code to be executed if condition3 is true
....
} else{
//code to be executed if all given conditions are false
}
Flowchart
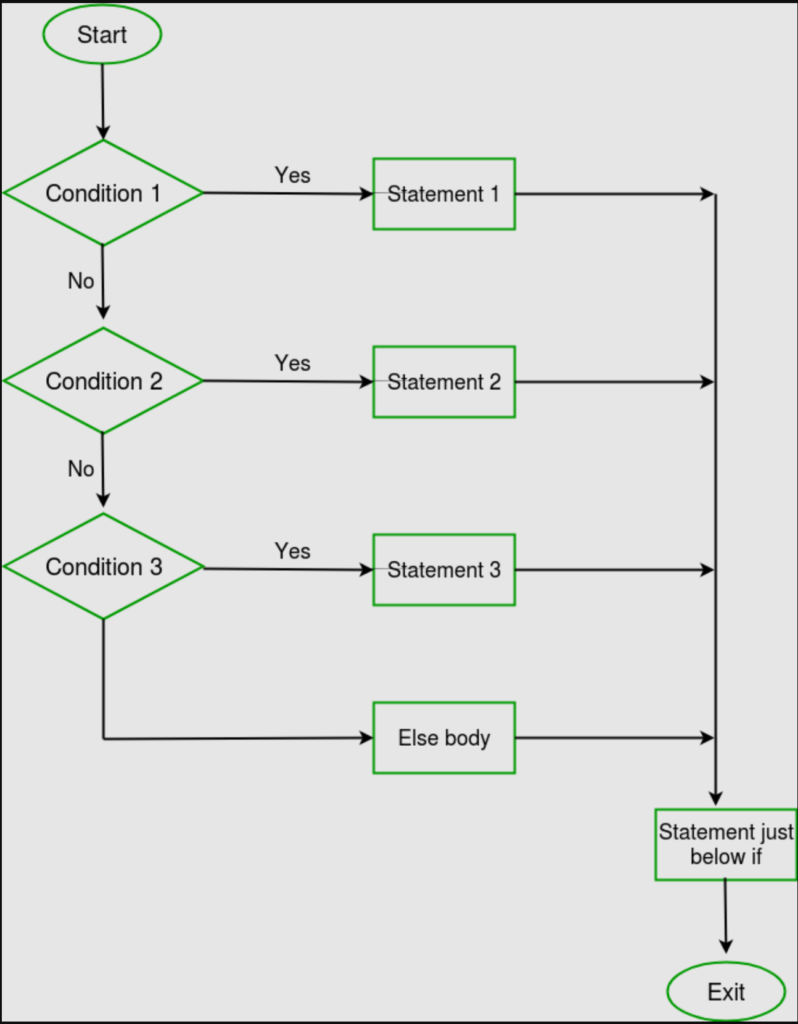
Example
<?php
$marks=91;
if ($marks<33){
echo "fail";
}
else if ($marks>=34 && $marks<50) {
echo "D grade";
}
else if ($marks>=50 && $marks<65) {
echo "C grade";
}
else if ($marks>=65 && $marks<80) {
echo "B grade";
}
else if ($marks>=80 && $marks<90) {
echo "A grade";
}
else if ($marks>=90 && $marks<100) {
echo "A+ grade";
}
else {
echo "Invalid input";
}
?>
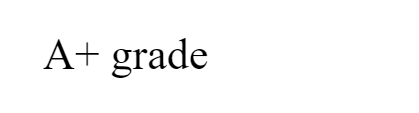
PHP nested if Statement
Nested if statements means an if block inside another if block.
The inner if statement executes only when specified condition in outer if statement is true.
If we insert a second if statement inside an if statement, we call it nested if statement or nesting the if statement.
Syntax
if (condition) {
//code to be executed if condition is true
if (condition) {
//code to be executed if condition is true
}
}
Flowchart
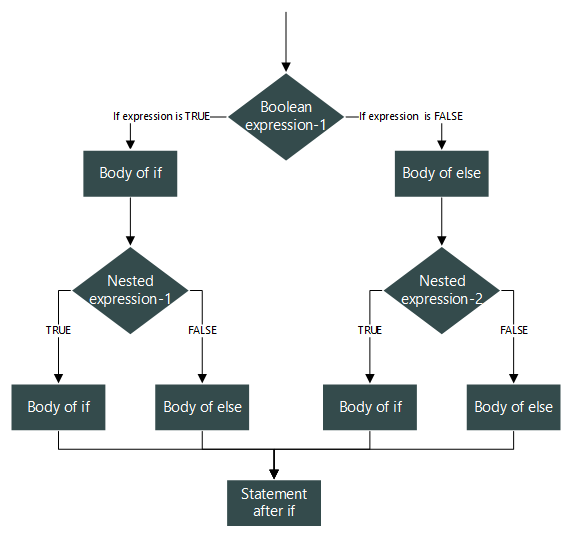
Example
<?php
$age = 21;
$nationality = "Indian";
//applying conditions on nationality and age
if ($nationality == "Indian")
{
if ($age >= 18) {
echo "Eligible to give vote";
}
else {
echo "Not eligible to give vote";
}
}
?>
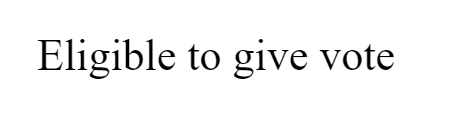