The continue statement is one of the looping control keywords in PHP. It skips one iteration in the loop (rest of the statements in current iteration of loop are skipped after continue statement ), if a specified condition occurs, and continues with the next iteration in the loop.
When the keyword continue executed inside a loop the control automatically passes to the beginning of loop.
The continue statement can be used with all types of loops such as – for, while, do-while, and foreach loop.
Syntax
jump-statement;
continue;
Flowchart
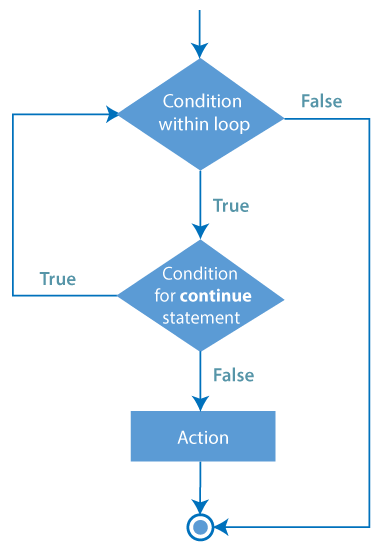
Example
PHP Continue Example with for loop
In below example, we will print only those values of i and j that are same and skip others.
<?php
//outer loop
for ($i =1; $i<=3; $i++) {
//inner loop
for ($j=1; $j<=3; $j++) {
if (!($i == $j) ) {
continue; //skip when i and j does not have same values
}
echo $i.$j;
echo "</br>";
}
}
?>

PHP continue Example in while loop
In below example, we will print the even numbers between 1 to 20.
<?php
//php program to demonstrate the use of continue statement
echo "Even numbers between 1 to 20: </br>";
$i = 1;
while ($i<=20) {
if ($i %2 == 1) {
$i++;
continue; //here it will skip rest of statements
}
echo $i;
echo " ";
$i++;
}
?>

PHP continue Example with array of string
<?php
/*The following example prints the value of array elements except those for which the specified condition is true and continue statement is used.*/
$number = array ("One", "Two", "Three", "Stop", "Four");
foreach ($number as $element) {
if ($element == "Stop") {
continue;
}
echo "$element ";
}
?>

PHP continue Example with optional argument
continue
accepts an optional numeric argument which tells it how many levels of enclosing loops it should skip to the end of. The default value is 1
, thus skipping to the end of the current loop.
The numeric value describes how many nested structures it will exit.
<?php
//outer loop
for ($i =1; $i<=3; $i++) {
//inner loop
for ($j=1; $j<=3; $j++) {
if (($i == $j) ) { //skip when i and j have same values
continue 1; //exit only from inner for loop
}
echo $i.$j;
echo "</br>";
}
}
?>
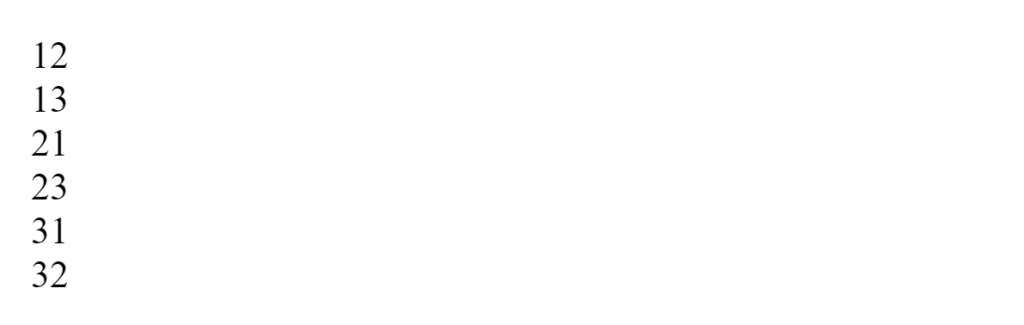