The break keyword immediately ends the execution of the loop or switch structure. It ends execution of the current for, foreach, while, do-while or switch structure.
If you use break inside inner loop, it breaks the execution of inner loop only. The break statement is one of the looping control keywords in PHP.
The break statement can be used in all types of loops such as while, do-while, for, foreach loop, and also with switch case.
Syntax
jump statement;
break;
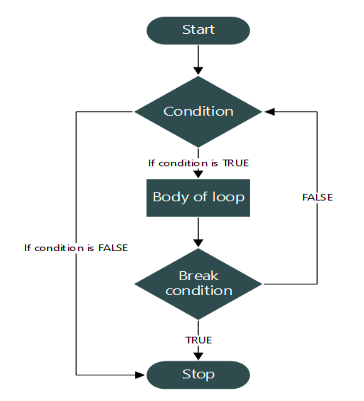
The break keyword immediately ends the execution of the current structure.
Examples
PHP Break: inside loop
Below is a example to break the execution of for loop if value of i is equal to 5.
<?php
for($i=1;$i<=10;$i++){
echo "$i";
if($i==5){
break;
}
}
?>
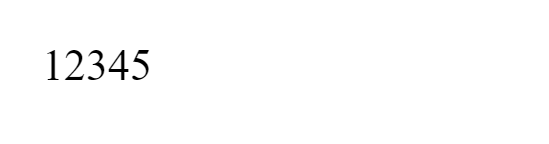
PHP Break: inside inner loop
In below example PHP break statement breaks the execution of inner loop only.
<?php
for($i=1;$i<=3;$i++){
for($j=1;$j<=3;$j++){
echo "$i $j<br/>";
if($i==2 && $j==2){
break;
}
}
}
?>
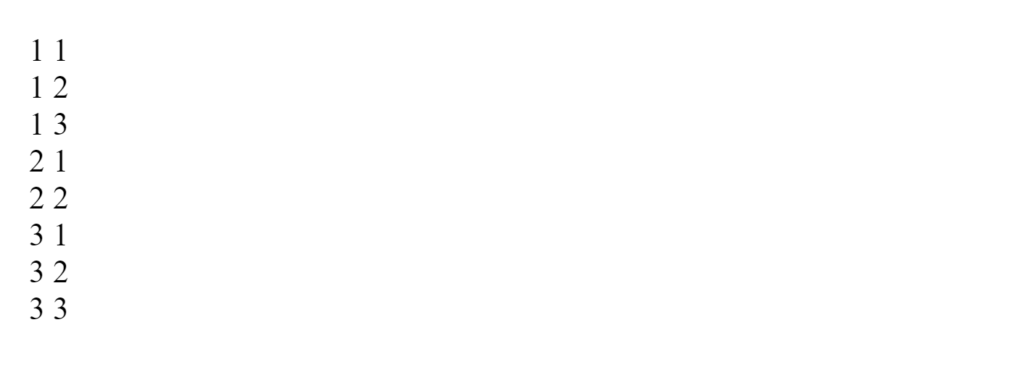
PHP Break: using optional argument
The PHP break keyword accepts an optional numeric argument, which describes how many nested structures it will exit. Its default value is 1, that means immediately exits from the enclosing structure.
<?php
$i = 0;
while (++$i) {
switch ($i) {
case 5:
echo "At matched condition i = 5<br />\n";
break 1; // Exit only from the switch.
case 10:
echo "At matched condition i = 10; quitting<br />\n";
break 2; // Exit from the switch and the while.
default:
break;
}
}?>

The PHP break statement breaks the flow of switch case also. If you don’t use break statement in switch case then it will check all cases even if match is found.
PHP Break: with array of string
<?php
//declare an array of string
$number = array ("One", "Two", "Three", "Stop", "Four");
foreach ($number as $element) {
if ($element == "Stop") {
break;
}
echo "$element </br>";
}
?>
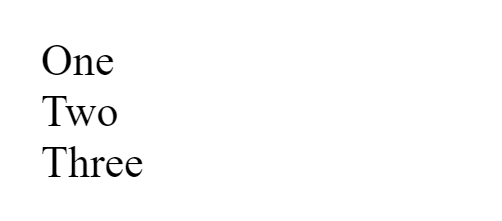