Arrow function does not have its own this
.
Whenever you call this
, it refers to its parent scope.
Inside a regular function, this keyword refers to the function where it is called.
With arrow functions the this
keyword always represents the object that defined the arrow function.
this
represents an object that executes the current function.
Example
Inside a regular function
function Person() {
this.name = 'Jack',
this.age = 25,
this.sayName = function () {
// this is accessible
console.log(this.age);
function innerFunc() {
// this refers to the global object
console.log(this.age);
console.log(this);
}
innerFunc();
}
}
let x = new Person();
x.sayName();
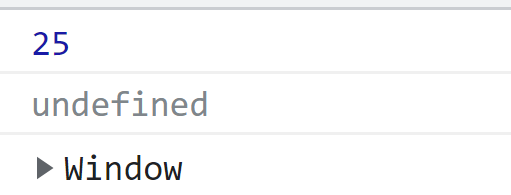
- Here, In above example
this.age
insidethis.sayName()
is accessible becausethis.sayName()
is the method of an object. - However,
innerFunc()
is a normal function andthis.age
is not accessible becausethis
refers to the global object (Window object in the browser). - Therefore,
this.age
inside theinnerFunc()
function givesundefined
.
Inside an arrow function
function Person() {
this.name = 'Jack',
this.age = 25,
this.sayName = function () {
console.log(this.age);
let innerFunc = () => {
console.log(this.age);
}
innerFunc();
}
}
const x = new Person();
x.sayName();
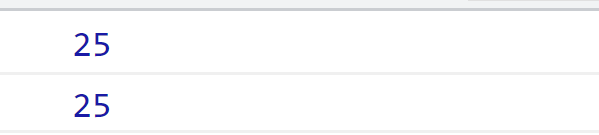
- Here in above example, the
innerFunc()
function is defined using the arrow function. - Inside the arrow function,
this
refers to the parent’s scope. - Therefore,
this.age
gives 25.