JavaScript comments are used to explain JavaScript code. It makes JavaScript code more readable. It can also be used to prevent execution, when testing alternative code.
Types of JavaScript Comments
There are two types of comments in JavaScript.
- Single-line Comment
- Multi-line Comment
This lesson will teach you how to create two types of comments in JavaScript: single line comments and multi-line comments.
We normally use them to describe how and why the code works.
Good comments allow us to maintain the code well, come back to it after a delay and use it more effectively.
Single Line Comments
It is represented by double forward slashes (//).
Single line comments start with //
. When you place these two slashes, all text to the right of them will be ignored, until the next line.
Any text between //
and the end of the line will not be executed.
Multi-line Comments
Multi-line comments start with /*
and end with */
.
Multi-line Comment Syntax
/* your comment here */
Any text between /*
and */
will be ignored by JavaScript.
JavaScript Comment example
<!DOCTYPE html>
<html>
<head>
<title>
JavaScript Comments
</title>
<script>
/* Script to get two input from user
and add them */
function add() {
// Declare three variable
var x, y, z;
/* Input the two nos. num1, num2
Input num1 and store it in x
Input num2 and store it in y
The sum is stored in a variable z*/
x = Number(document.getElementById("num1").value);
y = Number(document.getElementById("num2").value);
z = x + y; ;//It adds values of x and y variable
document.getElementById("sum").value = z;
}
// This is single line comment.
/* This is multi line comment.
This will not be displayed */
</script>
</head>
<body>
Enter the First number : <input id="num1"><br><br>
Enter the Second number: <input id="num2"><br><br>
<button onclick="add()">
Sum
</button>
<input id="sum">
</body>
</html>
Output of HTML Comment example
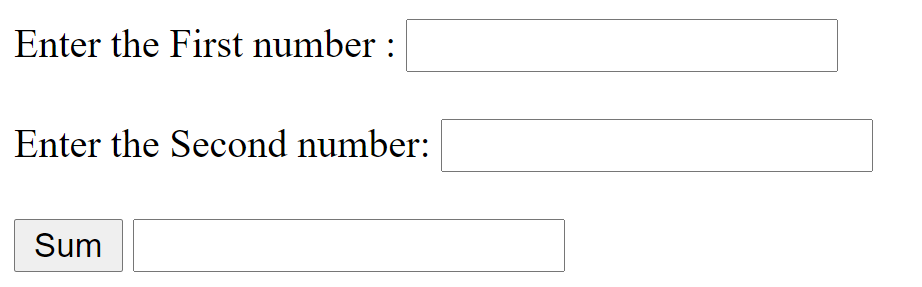
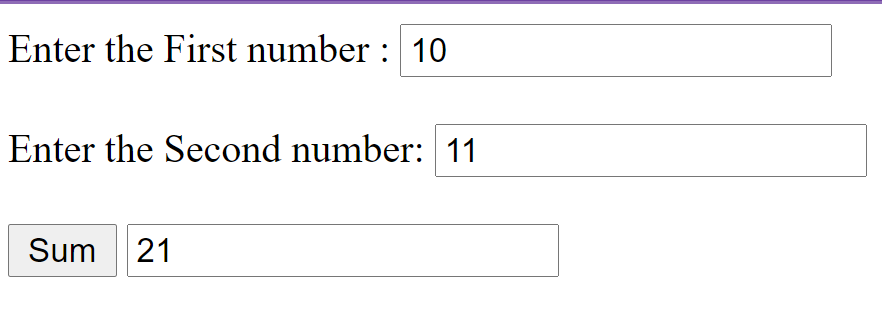
Advantages of JavaScript comments
- To make code easy to understand.
- It can also be used to avoid the code being executed. This is useful if you want to debug code. Commenting out code allows you to stop a line or multiple lines of code from running without removing the code from your program entirely.
Remember that commented code will never execute.
Every developer writes comments differently, but the general rule is that comments should be used to explain the intent behind your code.
In this tutorial, we discussed the basics of commenting and then explored how to write a comment in JavaScript.
Now you’re ready to write comments in JavaScript like an expert!