JavaScript Array values() Method creates a new array iterator object that carries the values specified at each array index.
The JavaScript Array values() method returns a new Array Iterator object that contains the values for each index in the array.
The values()
method does not change the original array.
The values()
method does not ignore empty array elements.
Syntax
array.values();
The values()
method does not have any parameters.
Return value
Returns a new Array
iterator object.
Examples
const array1 = ['a', 'b', 'c'];
const iterator = array1.values();
for (const value of iterator) {
console.log(value);
}
// expected output: "a"
// expected output: "b"
// expected output: "c"
const languages = ["JavaScript", "Java", , "C++", "Python", "Lua"];
let iterator = languages.values();
// using .next() with iterator object
console.log(iterator.next()); // { value: 'JavaScript', done: false }
console.log(iterator.next().value); // Java
console.log("Remaining:")
for (let value of iterator) {
console.log(value);
}
console.log(iterator.next()) // { value: undefined, done: true }
If the next() method use exceeds the given array length, it will return an ‘undefined’ value.
<html>
<head> <h5> Javascript Array Methods </h5> </head>
<body>
<script>
var arr=["John","Tom","Sheero","Romy","Tomy"]; //Initialized array
var itr=arr.values();
document.write("The array elements are: <br>");
document.write("<br>"+itr.next().value); //returns value at index 0.
document.write("<br>"+itr.next().value); //returns value at index 1
document.write("<br>"+itr.next().value); //returns value at index 2
document.write("<br>"+itr.next().value); //returns value at index 3
document.write("<br>"+itr.next().value); //returns value at index 4
document.write("<br>"+itr.next().value); //returns undefined
</script>
</body>
</html>
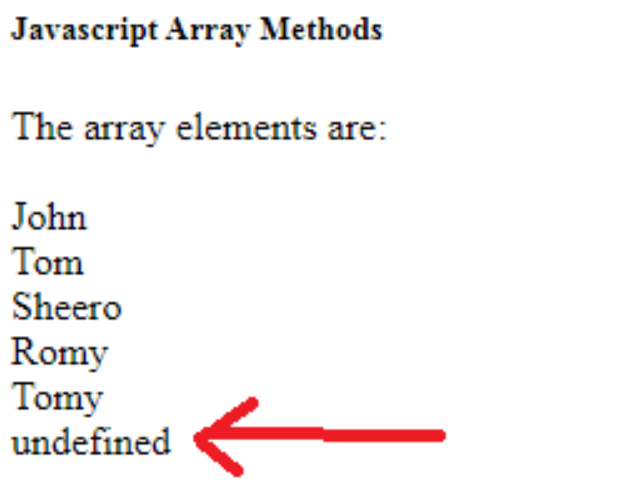
Iteration using for…of loop
const arr = ['a', 'b', 'c', 'd', 'e'];
const iterator = arr.values();
for (let letter of iterator) {
console.log(letter);
} //"a" "b" "c" "d" "e"