The concept of default parameters is a new feature introduced in the ES6 version of JavaScript. This allows us to give default values to function parameters.
If a function in JavaScript is called with missing arguments (less than declared), the missing values are set to undefined
.
Default function parameters allow formal parameters to be initialized with default values if no value or undefined is passed.
In this tutorial, you will learn about JavaScript default parameters with the help of examples.
Example
function say(message='Hi') {
console.log(message);
}
say(); // 'Hi'
say('Hello') // 'Hello'
The default value of the message
paramater in the say()
function is 'Hi'
.
Example-2
function sum(x = 3, y = 5) {
// return sum
return x + y;
}
console.log(sum(15, 15)); // 30
console.log(sum(10)); // 15
console.log(sum()); // 8
In the above example, the default value of x
is 3 and the default value of y
is 5.
sum(15, 15)
– When both arguments are passed,x
takes 15 andy
takes 15.sum(10)
– When 10 is passed to thesum()
function,x
takes 10 andy
takes default value 5.sum()
– When no argument is passed to the sum() function,x
takes default value 3 andy
takes default value 5.
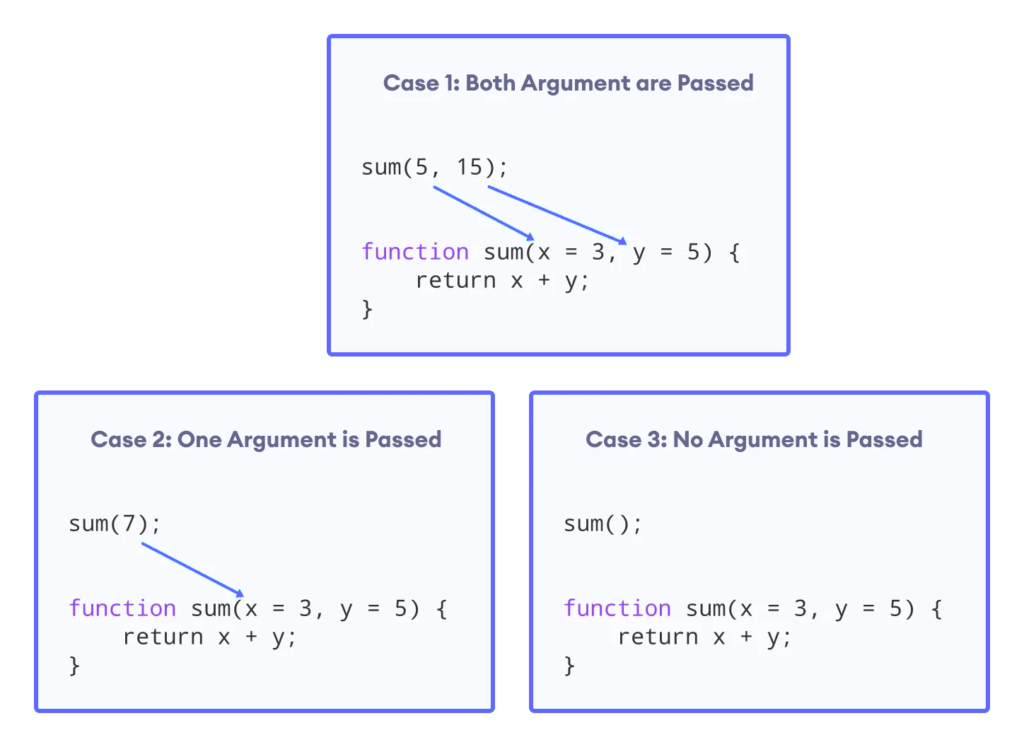
Arguments vs. Parameters
Parameters are what you specify in the function declaration whereas the arguments are what you pass to the function.
Example
function add(x, y) {
return x + y;
}
add(100,200);
In the above example, the x
and y
are the parameters of the add()
function, and the values passed to the add()
function 100
and 200
are the arguments.