JavaScript Array entries() Method returns an Array Iterator object with key/value pairs.
The entries()
method does not change the original array.
Contents
hide
Syntax
array.entries()
Parameters
It does not have any parameters.
Return Value
It returns the newly created array iterator object.
The iterator object represents each element of the array with keys assigned to them. A key represents the index number carrying an item as its value.
Examples
const languages = ["JavaScript", "Java", "C", "C++", "Python", "Lua"];
let iterator = languages.entries();
for (let entry of iterator) {
console.log(entry);
}
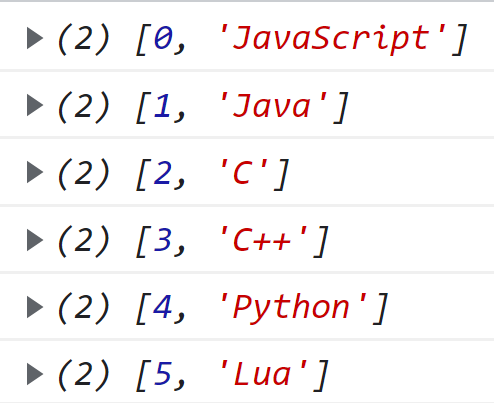
const array1 = ['a', 'b', 'c'];
const iterator1 = array1.entries();
console.log(iterator1.next().value);
// expected output: Array [0, "a"]
console.log(iterator1.next().value);
// expected output: Array [1, "b"]
const a = ['a', 'b', 'c'];
for (const [index, element] of a.entries())
console.log(index, element);
// 0 'a'
// 1 'b'
// 2 'c'
var a = ['a', 'b', 'c'];
var iterator = a.entries();
for (let e of iterator) {
console.log(e);
}
// [0, 'a']
// [1, 'b']
// [2, 'c']