This is part 4 of HTML Form series. You can read previous parts here. In this part you will learn about Submit button control and <fieldset> element.
<input type=”submit”> is used to add a submit button on web page. When user clicks on submit button, then the form gets submitted to the server. The form-handler is typically a server page with a script for processing the input data. The form-handler is specified in the form’s action
attribute.
Syntax
<input type="submit" value="submit">
- The type = submit , specifies that it is a submit button.
- The value attribute can be any text which will be shown on button. If you don’t specify a
value
, the button will have a default label, chosen by the user agent.
Example
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Submit button control example</title>
</head>
<body>
<form>
<label for="name">Enter name</label><br>
<input type="text" id="name" name="name"><br>
<label for="pass">Enter Password</label><br>
<input type="Password" id="pass" name="pass"><br>
<input type="submit" value="submit">
</form>
</body>
</html>
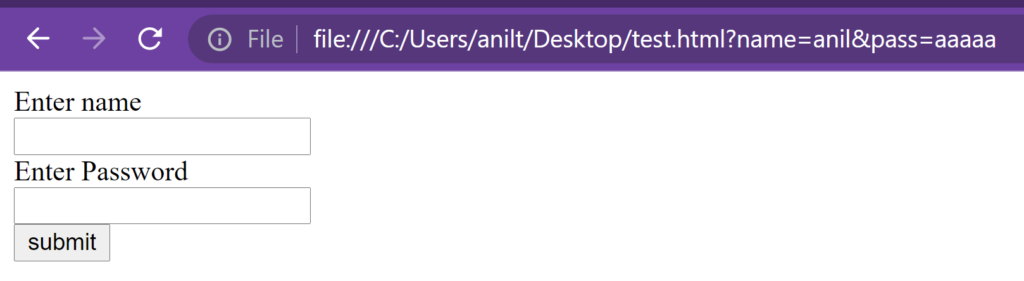
HTML <fieldset> element
The <fieldset> element in HTML is used to group the related information of a form. It is used with <legend> element which provide caption for the grouped elements.
The <fieldset>
tag draws a box around the related elements.
Example
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>fieldset example</title>
</head>
<body>
<form>
<fieldset>
<legend>User Information:</legend>
<label for="name">Enter name</label><br>
<input type="text" id="name" name="name"><br>
<label for="pass">Enter Password</label><br>
<input type="Password" id="pass" name="pass"><br>
<input type="submit" value="submit">
</fieldset>
</form>
</body>
</html>
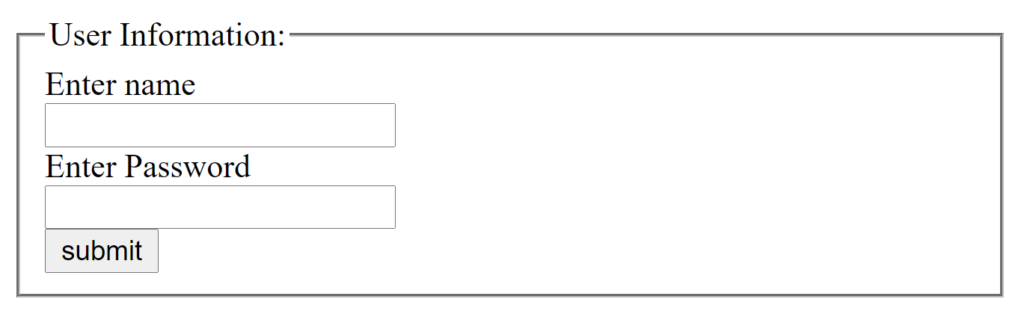
disabled
attribute of fieldset
This is a Boolean attribute. If it is set, all form controls that are descendants of the <fieldset>
, are disabled, meaning they are not editable and won’t be submitted along with the <form>
.
They won’t receive any browsing events, like mouse clicks or focus-related events. By default browsers display such controls grayed out.