This is part 2 of HTML Form series. You can read first part here. In this part you will learn about <textarea>, <label> and password field in forms.
<textarea> element of HTML form
The <textarea> tag in HTML is used to insert multiple-line text in a form. This is used when the user is required to give details that may be longer than a single sentence.
The size of <textarea> can be defined by using “rows” or “cols” attribute or by CSS. The rows
attribute specifies the visible number of lines in a text area. The cols
attribute specifies the visible width of a text area.
Example
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>HTML form textarea example</title>
</head>
<body>
<form>
Enter your address:<br>
<textarea rows="2" cols="20"></textarea>
</form>
</body>
</html>
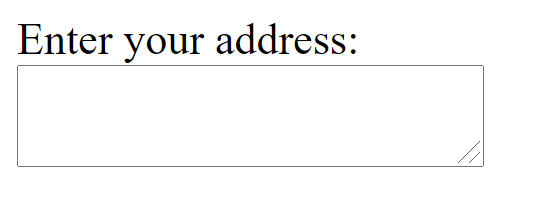
<label> element of HTML form
The <label>
HTML element represents a caption for an item in a user interface.
The <label>
element defines a label for several form elements. When a user clicks or touches/taps a label, the browser passes the focus to its associated input control. It is more worthy with touchscreens.
The for
attribute of the <label>
tag should be equal to the id
attribute of the <input>
element to bind them together.
Example
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>HTML form label example</title>
</head>
<body>
<form>
<label for="firstname">First Name: </label> <br>
<input type="text" id="firstname" name="firstname" /> <br>
<label for="lastname">Last Name: </label><br>
<input type="text" id="lastname" name="lastname" /> <br>
</form>
</body>
</html>
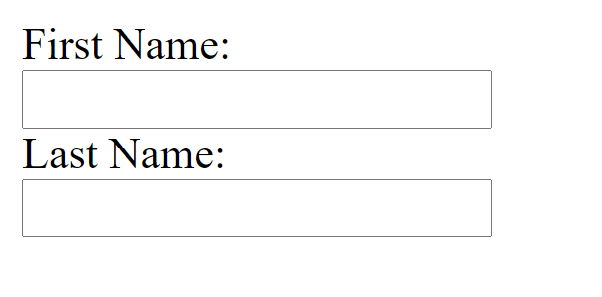
In above example tap on label “First Name” or “Last Name” as soon as you touch on label it will shift focus on its associated input control.
HTML Password Field Control
The password is not visible to the user in password field control. This is also a single-line text input but it masks the character as soon as a user enters it.
Password fields are a type of text field in which the text entered is masked using asterisk or dots for prevention of user identity from another person who is looking onto the screen. It also created using HTMl <input> tag by setting type attribute to password.
Example
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>HTML form password example</title>
</head>
<body>
<form>
<label for="password">Password: </label> <br>
<input type="password" id="password" name="password" />
</form>
</body>
</html>
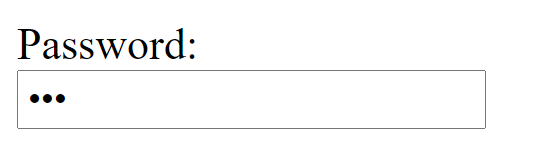